Preventing public access to buckets and files in Google Cloud Storage using Python
Start your free 7-days trial now!
Prerequisites
To follow along with this guide, please make sure to have:
created a service account and downloaded the private key (JSON file) for authentication (please check out my detailed guide)
installed the Python client library for Google Cloud Storage:
pip install --upgrade google-cloud-storage
Preventing public access to buckets and files in Google Cloud Storage
To prevent public access to buckets and files in Google Cloud Storage using Python, enable the Prevent Public Access option via the iam_configuration
property of the bucket:
from google.cloud.storage.constants import PUBLIC_ACCESS_PREVENTION_ENFORCEDfrom google.cloud import storage
# Authenticate ourselves using the primary key of our service accountpath_to_private_key = './gcs-project-354207-099ef6796af6.json'client = storage.Client.from_service_account_json(json_credentials_path=path_to_private_key)# The target bucketbucket = client.get_bucket('test-bucket-skytowner')bucket.iam_configuration.public_access_prevention = PUBLIC_ACCESS_PREVENTION_ENFORCED# Apply the changesbucket.patch()
Here, note the following:
we had to import the
PUBLIC_ACCESS_PREVENTION_ENFORCED
constant, which is actually just a string of value'enforced'
.all the files within the bucket will no longer be publicly accessible
Note that we can replace PUBLIC_ACCESS_PREVENTION_ENFORCED
with PUBLIC_ACCESS_PREVENTION_INHERITED
in the above code snippet to disable Public Access Prevention programmatically.
To confirm that the Public Access Prevention is now turned on for the bucket, head over to the web console for GCS, and click on the bucket (test-bucket-skytowner
in our case) and then click on the PERMISSIONS tab:
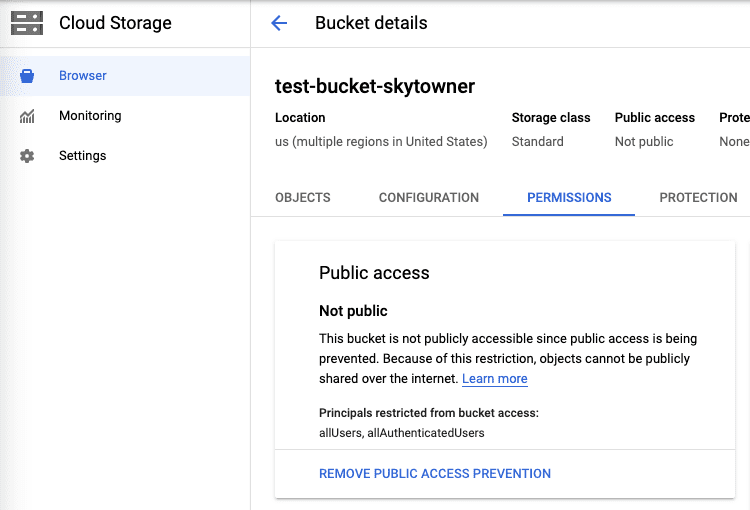
Notice how the bucket (and all the files within this bucket) are not publicly accessible, and that there is now an option to REMOVE PUBLIC ACCESS PREVENTION.